New Node.js Fluent API for DSE Graph
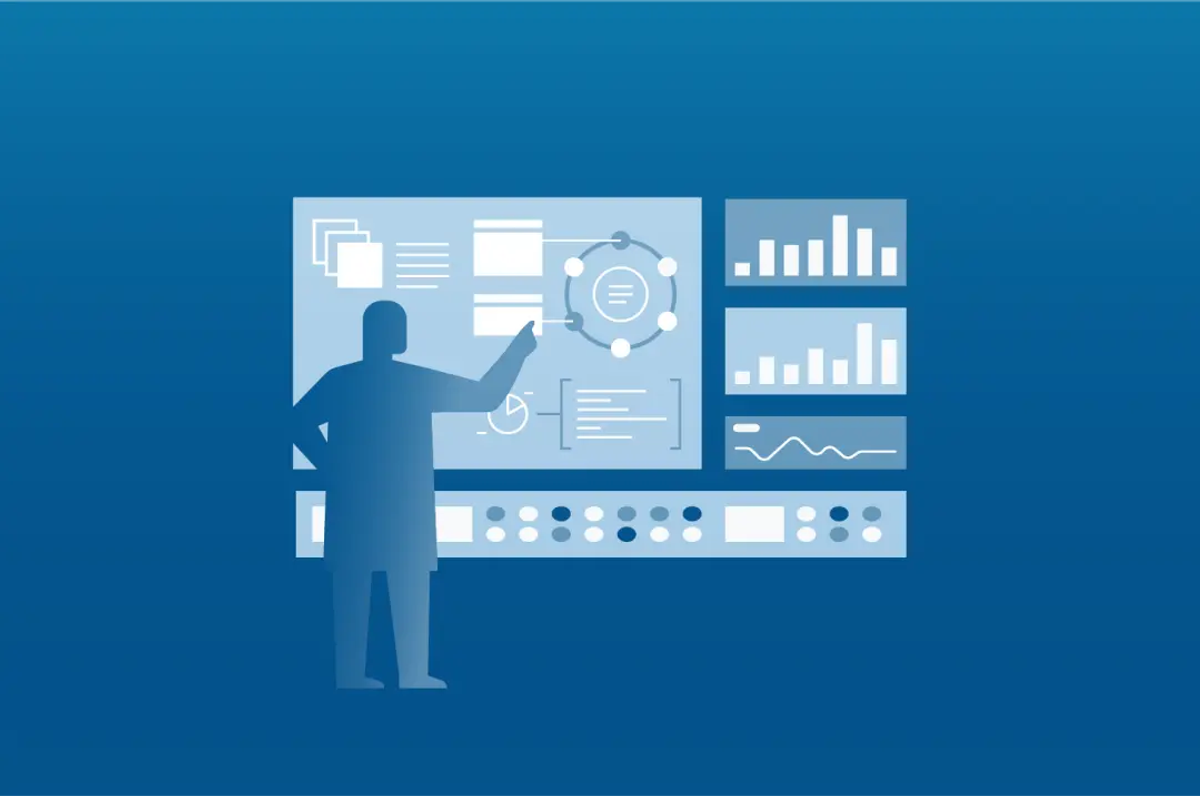
We've recently released the Node.js Fluent API for interacting with DSE Graph using Apache TinkerPop™ Gremlin-JavaScript.
Let's see how this new library can help you write Gremlin traversals from your JavaScript code.
Gremlin JavaScript Variant and the DataStax Driver Integration
Gremlin is a functional language used to define graph traversals. There's a single Gremlin language with different variants based on the native programming language, allowing users to write their traversals alongside their application code and benefit from the advantages afforded by the host language and its tooling.
In the case of the DataStax Node.js Fluent API, we are using Gremlin-JavaScript language variant. You can read more information regarding Gremlin and GLVs on this blog post by Kevin Gallardo.
The Node.js Fluent API for DSE Graph leverages the high-level client driver features of the DataStax Enterprise Node.js Driver, including:
- Automatic cluster discovery
- Built-in load-balancing features
- Datacenter awareness
- Failure recovery policies
- Speculative executions
- Enterprise-grade client authentication
It was previously only possible to use the DataStax Node.js Driver to execute string representations of groovy gremlin traversals on DSE Graph. With this new package you can write your graph traversals in JavaScript.
Getting Started
Add the package reference to your project file and obtain it from npm registry:
npm install dse-graph
Import the following modules.
const dse = require('dse-driver'); const dseGraph = require('dse-graph');
To execute graph traversals, you will need a DSE Driver Client instance that represents a pool of connections to your DSE cluster.
const client = new dse.Client({ contactPoints: ['host1', 'host2'], profiles: [ dseGraph.createExecutionProfile('default', { graphOptions: { name: 'my_graph' } }) ] });
Use your Client
instances to obtain GraphTraversalSource
instances.
const g = dseGraph.traversal(client);
The dseGraph.traversal()
method supports specifying graph options along with the execution profile. When no execution profile is provided, 'default' is used.
const g = dseGraph.traversal(client, { executionProfile: 'other-profile' });
A GraphTraversalSource (g)
from Gremlin-JavaScript can be used (and reused) to build traversals.
const traversal = g.V().has('name', 'matt').out('knows').values('name');
You can execute the traversal by calling toList()
, which returns a Promise
resolving to an Array
of values.
traversal.toList().then(friendNames => console.log(friendNames));
On async functions, you can await on the returned Promise
.
const friendNames = await traversal.toList();
console.log(friendNames);
Visit the Getting Started Guide for more code examples and information.
Batching Traversals
DSE Graph supports batching multiple graph updates into a single transaction. All mutations included in a batch will be applied if the execution completes successfully or none of them if any of the operations fail.
In a nutshell, after creating an Execution Profile with this package, you can use the DSE driver to execute a batch of traversals.
const batch = [
g.addV('person').property('name', 'Matt').property('age', 12),
g.addV('person').property('name', 'Olivia').property('age', 8),
g.V().has('name', 'Matt').addE('knows').to(__.V().has('name', 'Olivia'))
];
const query = dseGraph.queryFromBatch(batch);
// Execute the batch. Note that an Execution Profile is needed
client.executeGraph(query);
You can continue reading about batching on the documentation.
Wrapping Up
The documentation for the DataStax Node.js Driver Fluent API for DSE Graph is located here.
Your feedback is important to us, you can use the following channels to send comments and/or questions:
- Mailing List: https://groups.google.com/a/lists.datastax.com/forum/#!forum/nodejs-driver-user
- Report issues on JIRA: https://datastax-oss.atlassian.net/browse/NODEJS/issues