DataStax Java Driver: 2.2.0-rc1 released!
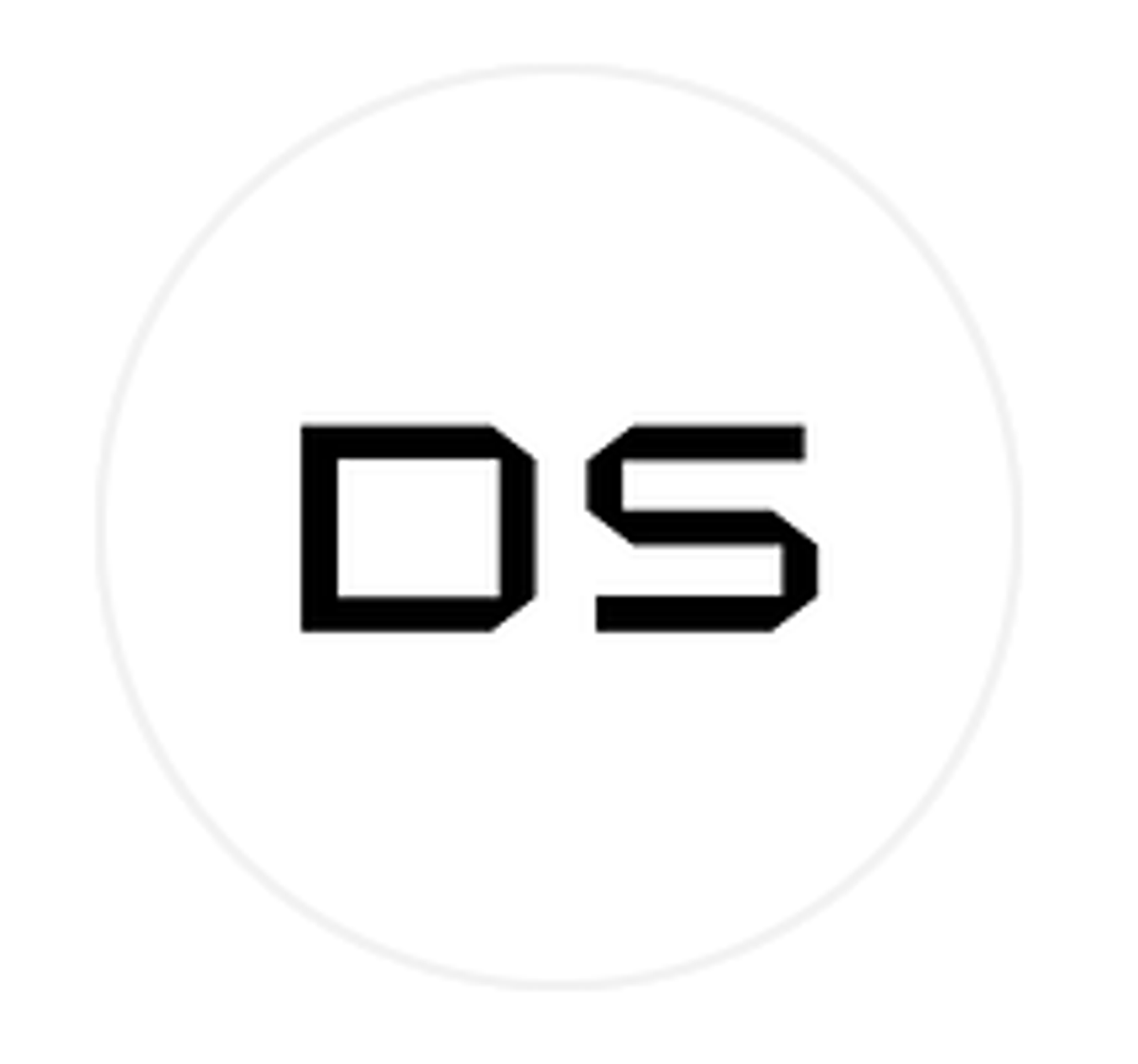
The Java driver team is pleased to announce the release of version 2.2.0-rc1, which brings parity with Cassandra 2.2. Here's what the new Cassandra features change for driver users:
- New CQL data types
SMALLINT
andTINYINT
DATE
andTIME
- New metadata for user-defined functions
- Unbound variables
- Query warnings
New CQL data types
Four new types were introduced:
SMALLINT
and TINYINT
These two integer types match Java's short
and byte
, so their usage should be quite straightforward:
1 2 3 4 5 6 7 |
|
There is one minor catch: Java's integer literals default to int
, which the driver serializes as CQLINT
s. So the following will fail:
1 2 3 |
|
The workaround is simply to coerce your arguments to the correct type:
1 |
|
DATE
and TIME
In previous versions, Cassandra only had TIMESTAMP
to represent date and time. The two new types add support for date-only and time-only values:
1 2 3 4 5 6 7 |
|
The getter for TIMESTAMP
used to be called getDate
, we renamed it to getTimestamp
; getDate
is now used for the DATE
type. This is obviously a breaking change when migrating from a previous driver version, but we think the consistency was worth it.
DATE
is modeled with a custom Java type called DateWithoutTime
(but the name is likely to change before 2.2 goes GA). This was not an easy decision to make, but we ruled out other possibilities:
- The new
java.time
types from Java 8 were not an option, because we want to keep compatibility with older JDKs. - We didn't want to bring in an additional dependency to Joda Time. And if we had, the logical thing would have been to migrate all time types to Joda, but its
LocalTime
type does not have nanosecond resolution.
We hope to introduce custom serializers soon (JAVA-721), so that users can use their preferred Java representations.
TIME
is modeled with a long
, which represents the number of nanoseconds since midnight.
New metadata for user-defined functions
Cassandra 2.2 introduces user-defined functions. Normal functions operate on individual rows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
Aggregates combine rows to compute a compound value:
1 2 3 4 5 6 7 8 9 |
|
From the client side, calling a user-defined function is not particularly different from a built-in one:
1 2 3 4 5 |
|
The most important addition to the driver is that functions are now exposed as metadata:
1 2 3 4 5 6 7 8 9 |
|
Note that, in order to retrieve a function or aggregate from a keyspace, you need to specify the argument types, to distinguish overloaded versions.
Unbound variables
In previous driver versions, you had to provide all variables in a bound statement, or it would get rejected. This is no longer the case:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
As you can see, unset values do not overwrite previously inserted ones.
Unset values are not always legal. Cassandra will throw an error if the resulting query is invalid:
1 2 3 |
|
Query warnings
Cassandra now sends warnings back with the response instead of just logging them server-side. The driver exposes them through ExecutionInfo. The easiest way to observe this is to simulate a large batch:
1 2 3 4 5 6 7 8 9 10 11 |
|
Getting the driver
As always, the driver is available from Maven and from our downloads server.
Please note that this is a release candidate and is not meant to be used in production. The public API is subject to change until the final release. If you are planning to upgrade soon, be sure to read our upgrade guide.
We're also running a platform and runtime survey to improve our testing infrastructure. Your feedback would be most appreciated.